There are lots of questions/posts about dealing with duplicate records in many SQL Server forums. Many of these questions are asked in “SQL Server Interviews” and many developers starting their carrier in database programming find it challenging to deal with. Here we will see how we can deal with such records.
Duplicate data is one of the biggest pain points in our IT industry, which various projects have to deal with. Whatever state-of-art technology and best practices followed, the big ERP, CRM, SCM and other inventory based database management projects ends up in having duplicate & redundant data. Duplicate data keeps on increasing by manual entries and automated data loads. Various data leads getting pumped into system’s databases without proper deduping & data cleansing leads to redundant data and thus duplicated record-sets.
Data cleansing requires regular exercise of identifying duplicates, validating and removing them. To minimize these type of scenarios various checks and filters should also be applied before loading new leads into the system.
Lets check this by a simple exercise how we can identify & remove duplicate data from a table:
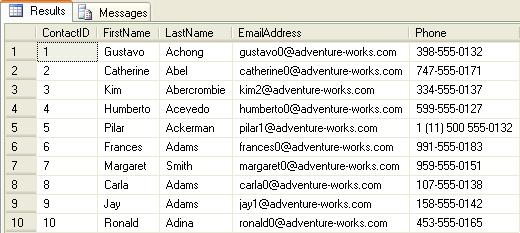


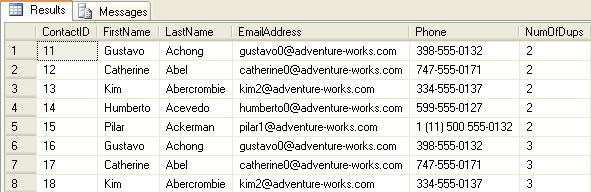
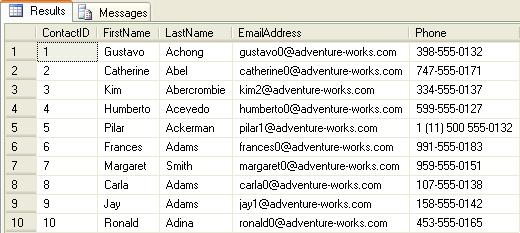

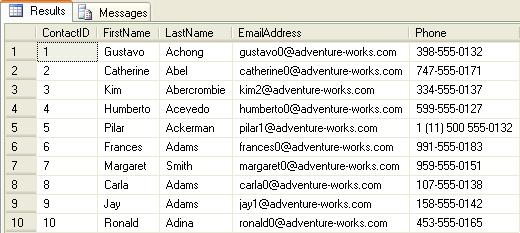

Referenced from : http://sqlwithmanoj.wordpress.com/category/sql-tips/
Duplicate data is one of the biggest pain points in our IT industry, which various projects have to deal with. Whatever state-of-art technology and best practices followed, the big ERP, CRM, SCM and other inventory based database management projects ends up in having duplicate & redundant data. Duplicate data keeps on increasing by manual entries and automated data loads. Various data leads getting pumped into system’s databases without proper deduping & data cleansing leads to redundant data and thus duplicated record-sets.
Data cleansing requires regular exercise of identifying duplicates, validating and removing them. To minimize these type of scenarios various checks and filters should also be applied before loading new leads into the system.
Lets check this by a simple exercise how we can identify & remove duplicate data from a table:
1 | USE [AdventureWorks] |
2 | GO |
3 |
4 | -- Insert some sample records from Person.Contact table of [AdventureWorks] database: |
5 | SELECT TOP 10 ContactID, FirstName, LastName, EmailAddress, Phone |
6 | INTO DupContacts |
7 | FROM Person.Contact |
8 |
9 | SELECT * FROM DupContacts |
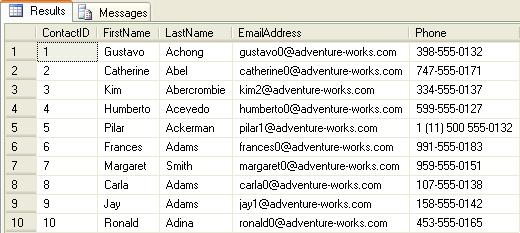
1 | -- Insert some duplicate records from the same list inserted above. |
2 | INSERT INTO DupContacts |
3 | SELECT TOP 50 PERCENT FirstName, LastName, EmailAddress, Phone |
4 | from DupContacts |
5 |
6 | SELECT * FROM DupContacts |

1 | -- Insert some more duplicate records from the same list inserted above. |
2 | INSERT INTO DupContacts |
3 | SELECT TOP 20 PERCENT FirstName, LastName, EmailAddress, Phone |
4 | from DupContacts |
5 |
6 | SELECT * FROM DupContacts |

01 | --// Identify Duplicate records & delete them:- |
02 |
03 | -- Method #1: by using ROW_NUMBER() function: |
04 | ; WITH dup as ( |
05 | SELECT ContactID, FirstName, LastName, EmailAddress, Phone, |
06 | ROW_NUMBER() OVER(PARTITION BY FirstName, LastName ORDER BY ContactID) AS NumOfDups |
07 | FROM DupContacts) |
08 | SELECT * FROM dup |
09 | WHERE NumOfDups > 1 |
10 | ORDER BY ContactID |
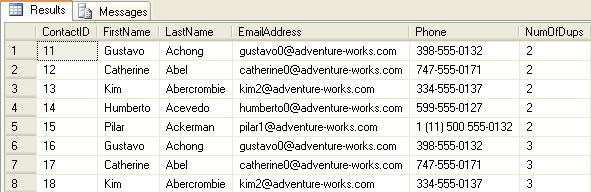
1 | -- Remove/Delete duplicate records: |
2 | ; WITH dup as ( |
3 | SELECT ContactID, FirstName, LastName, EmailAddress, Phone, |
4 | ROW_NUMBER() OVER(PARTITION BY FirstName, LastName ORDER BY ContactID) AS NumOfDups |
5 | FROM DupContacts) |
6 | DELETE FROM dup |
7 | WHERE NumOfDups > 1 |
8 |
9 | SELECT * FROM DupContacts |
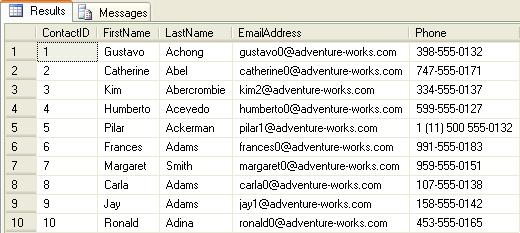
1 | -- Method #2: by using SELF-JOIN: |
2 | SELECT DISTINCT a.ContactID, a.FirstName, a.LastName, a.EmailAddress, a.Phone |
3 | FROM DupContacts a |
4 | JOIN DupContacts b |
5 | ON a.FirstName = b.FirstName |
6 | AND a.LastName = b.LastName |
7 | AND a.ContactID > b.ContactID |

1 | -- Remove/Delete duplicate records: |
2 | DELETE a |
3 | FROM DupContacts a |
4 | JOIN DupContacts b |
5 | ON a.FirstName = b.FirstName |
6 | AND a.LastName = b.LastName |
7 | AND a.ContactID > b.ContactID |
8 |
9 | SELECT * FROM DupContacts |
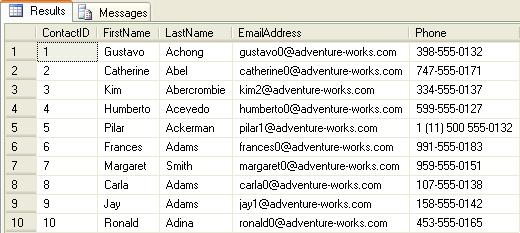
1 | -- Method #3: by using AGGREGATES & Sub-QUERY: |
2 | SELECT * FROM DupContacts |
3 | WHERE ContactID NOT IN ( SELECT MIN (ContactID) |
4 | FROM DupContacts |
5 | GROUP BY FirstName, LastName) |

1 | -- Remove/Delete duplicate records: |
2 | DELETE FROM DupContacts |
3 | WHERE ContactID NOT IN ( SELECT MIN (ContactID) |
4 | FROM DupContacts |
5 | GROUP BY FirstName, LastName) |
6 |
7 | SELECT * FROM DupContacts |
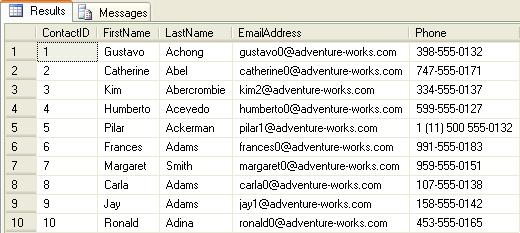
Referenced from : http://sqlwithmanoj.wordpress.com/category/sql-tips/
No comments:
Post a Comment